The solution of question 8
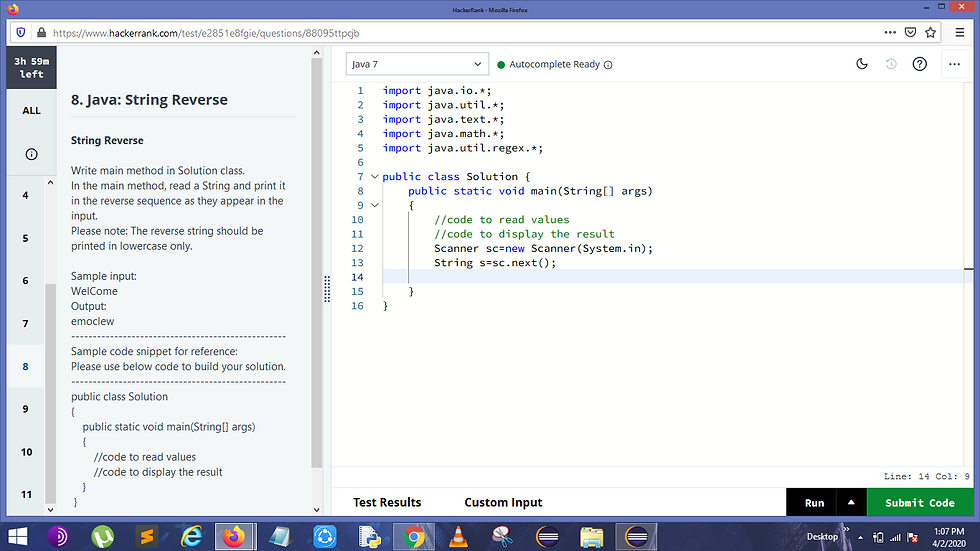
import java.io.*;
import java.util.*;
import java.text.*;
import java.math.*;
import java.util.regex.*;
public class Solution {
public static void main(String[] args)
{
//code to read values
//code to display the result
Scanner sc=new Scanner(System.in);
String s=sc.nextLine();
// System.out.println(s);
String s1=s.toLowerCase();
for (int i=s1.length()-1;i>=0;i--)
{
System.out.print(s1.charAt(i));
}
}
}
The solution of Question No.9
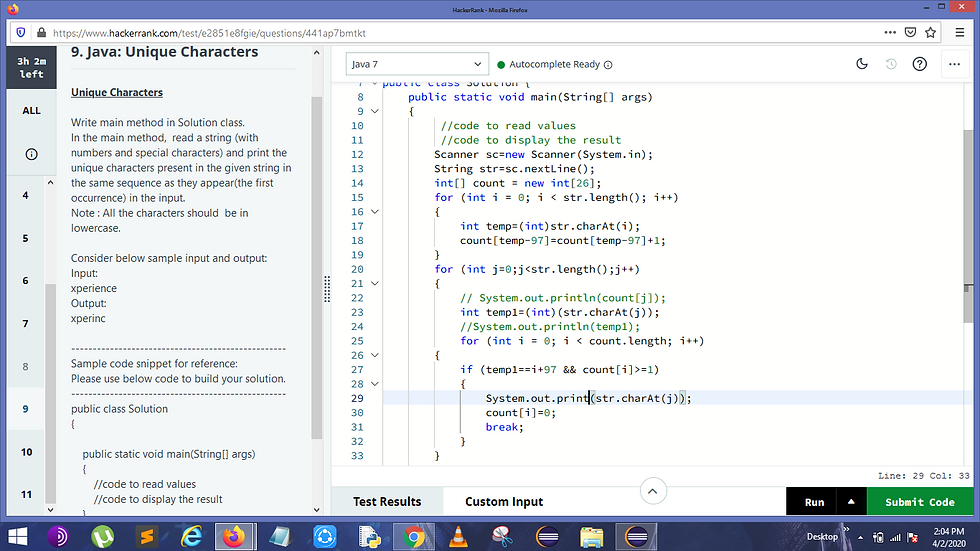
import java.io.*;
import java.util.*;
import java.text.*;
import java.math.*;
import java.util.regex.*;
public class Solution {
public static void main(String[] args)
{
//code to read values
//code to display the result
Scanner sc=new Scanner(System.in);
String str=sc.nextLine();
int[] count = new int[26];
for (int i = 0; i < str.length(); i++)
{
int temp=(int)str.charAt(i);
count[temp-97]=count[temp-97]+1;
}
for (int j=0;j<str.length();j++)
{
// System.out.println(count[j]);
int temp1=(int)(str.charAt(j));
//System.out.println(temp1);
for (int i = 0; i < count.length; i++)
{
if (temp1==i+97 && count[i]>=1)
{
System.out.print(str.charAt(j));
count[i]=0;
break;
}
}
}
// Set<Character> s= new HashSet<Character>();
// for (int i = 0; i < str.length(); i++)
// {
// s.add(str.charAt(i));
// }
// int arr[]=new int[26];
// s.toArray(arr);
// for (int i = 0; i < arr.length; i++)
// {
// System.out.println(arr[i]);
// }
// System.out.println(s);
}
}
The solution of Question No.10
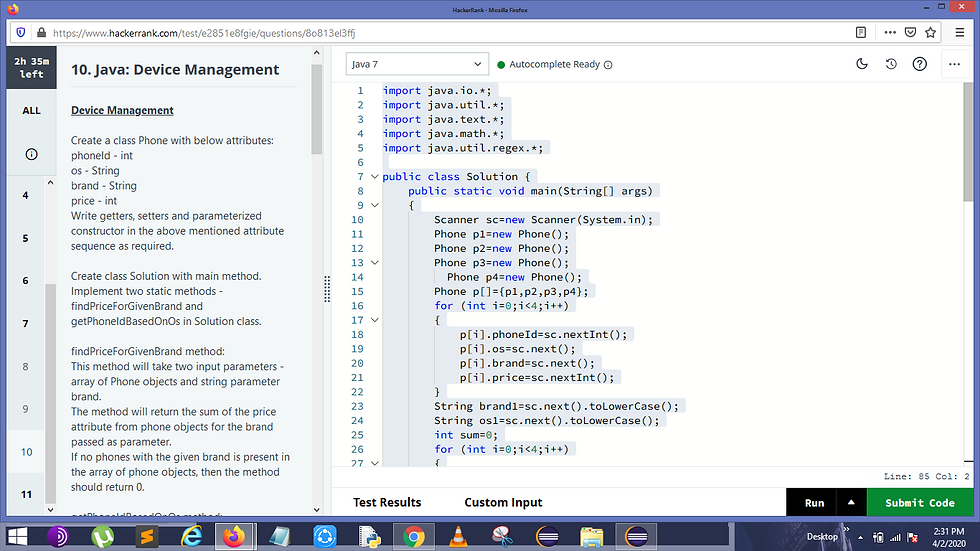
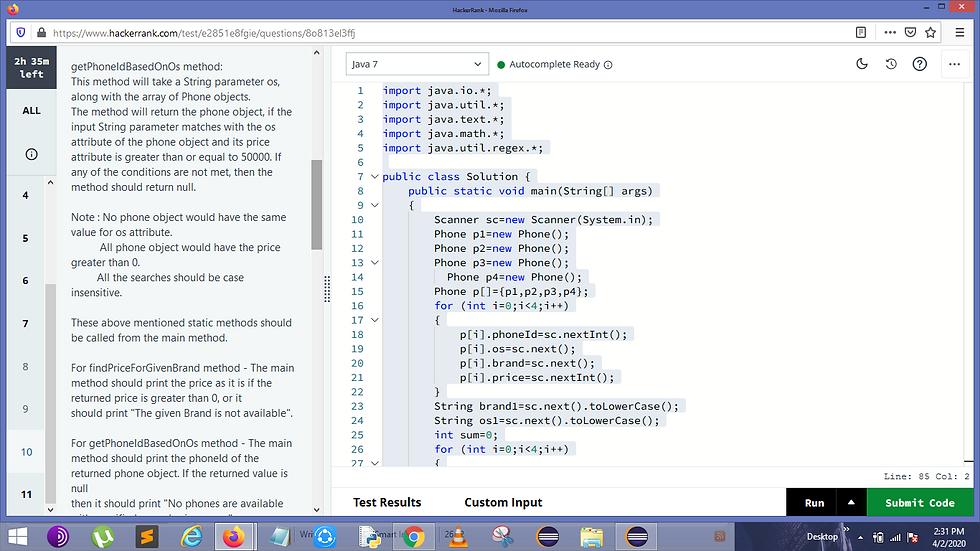
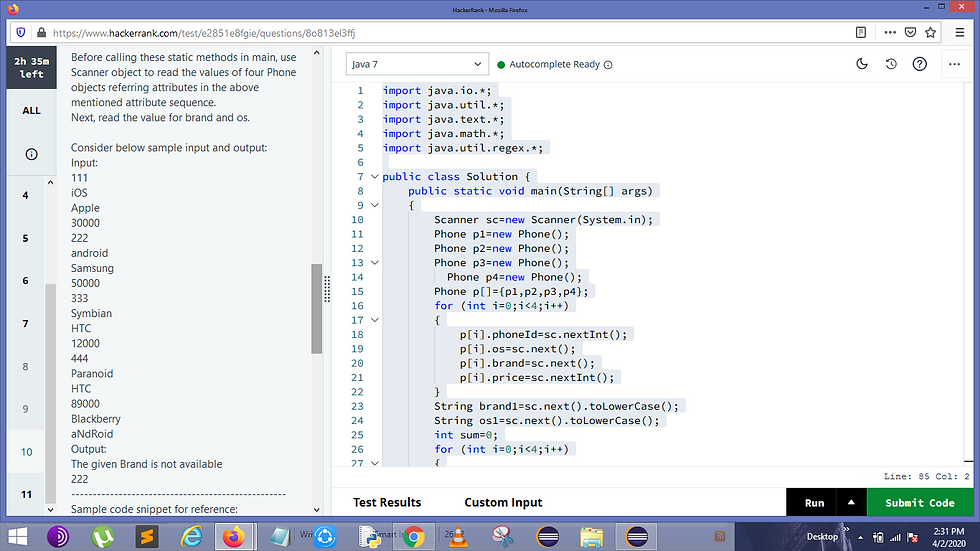
import java.io.*;
import java.util.*;
import java.text.*;
import java.math.*;
import java.util.regex.*;
public class Solution {
public static void main(String[] args)
{
Scanner sc=new Scanner(System.in);
Phone p1=new Phone();
Phone p2=new Phone();
Phone p3=new Phone();
Phone p4=new Phone();
Phone p[]={p1,p2,p3,p4};
for (int i=0;i<4;i++)
{
p[i].phoneId=sc.nextInt();
p[i].os=sc.next();
p[i].brand=sc.next();
p[i].price=sc.nextInt();
}
String brand1=sc.next().toLowerCase();
String os1=sc.next().toLowerCase();
int sum=0;
for (int i=0;i<4;i++)
{
// System.out.println(p[i].brand.toLowerCase()+" "+brand1+"ah");
if (p[i].brand.toLowerCase().equals(brand1))
{
sum=sum+p[i].price;
}
}
if (sum>0)
{
System.out.println(sum);
}
else
{
System.out.println("The given Brand is not available");
}
ArrayList <Integer>a=new ArrayList();
for (int i=0;i<4;i++)
{
if(p[i].os.toLowerCase().equals(os1) && p[i].price>=50000 )
{
a.add(p[i].phoneId);
}
}
if (a.size()==0)
{
System.out.println("No phones are available with specified os and price range");
}
else{
for (int k=0;k<a.size();k++)
{
System.out.println(a.get(k));
}
}
// public static int findPriceForGivenBrand(Phone[] phone, String brand)
// {
// //method logic
// }
// public static Phone getPhoneIdBasedOnOs(Phone[] phone, String os)
// {
// //method logic
// }
}
}
class Phone
{
int phoneId;
String os;
String brand;
int price;
//code to build Phone class
}
The solution of Question No.11


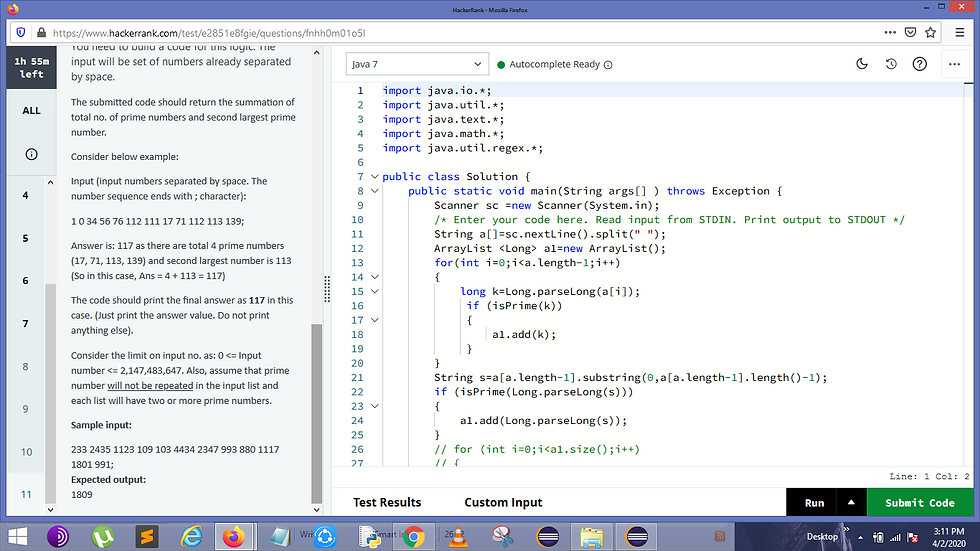
import java.io.*;
import java.util.*;
import java.text.*;
import java.math.*;
import java.util.regex.*;
public class Solution {
public static void main(String args[] ) throws Exception {
Scanner sc =new Scanner(System.in);
/* Enter your code here. Read input from STDIN. Print output to STDOUT */
String a[]=sc.nextLine().split(" ");
ArrayList <Long> a1=new ArrayList();
for(int i=0;i<a.length-1;i++)
{
long k=Long.parseLong(a[i]);
if (isPrime(k))
{
a1.add(k);
}
}
String s=a[a.length-1].substring(0,a[a.length-1].length()-1);
if (isPrime(Long.parseLong(s)))
{
a1.add(Long.parseLong(s));
}
// for (int i=0;i<a1.size();i++)
// {
// System.out.println(a1.get(i));
// }
Collections.sort(a1);
// for (int i=0;i<a1.size();i++)
// {
// System.out.println(a1.get(i));
// }
long sum=a1.size()+a1.get(a1.size()-2);
System.out.println(sum);
}
public static boolean isPrime(long k)
{
if (k==2)
{
return true;
}
boolean flag=true;
for (long j=2;j<k/2;j++)
{
if (k%j==0 && k!=1)
{
flag=false;
}
}
return flag;
}
}
Comentarios